Getting started
editGetting started
editThis page guides you through the installation process of the Node.js client, shows you how to instantiate the client, and how to perform basic Elasticsearch operations with it.
Requirements
editInstallation
editTo install the latest version of the client, run the following command:
npm install @elastic/elasticsearch
Refer to the Installation page to learn more.
Connecting
editYou can connect to the Elastic Cloud using an API key and the Elasticsearch endpoint.
const { Client } = require('@elastic/elasticsearch') const client = new Client({ node: 'https://...', // Elasticsearch endpoint auth: { apiKey: { // API key ID and secret id: 'foo', api_key: 'bar', } } })
Your Elasticsearch endpoint can be found on the My deployment page of your deployment:
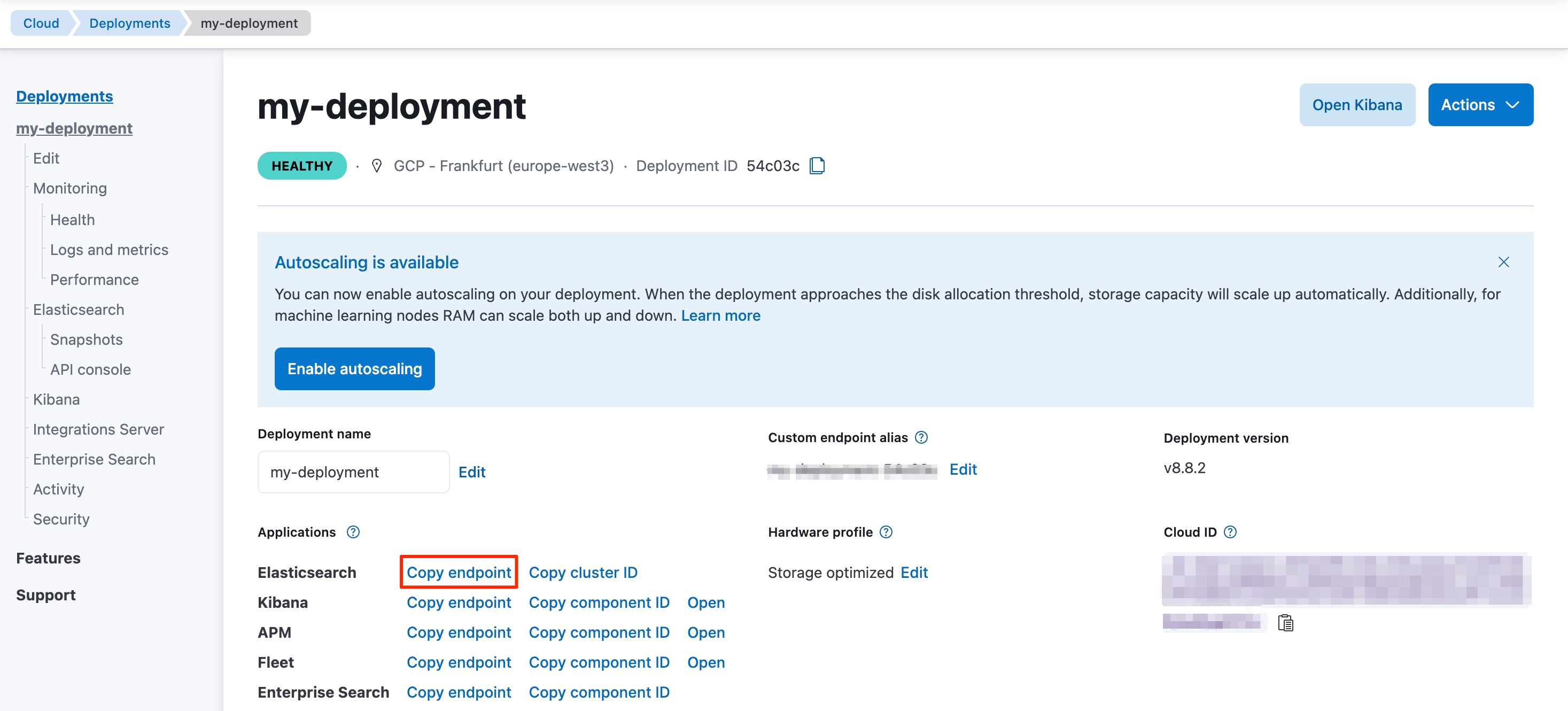
You can generate an API key on the Management page under Security.
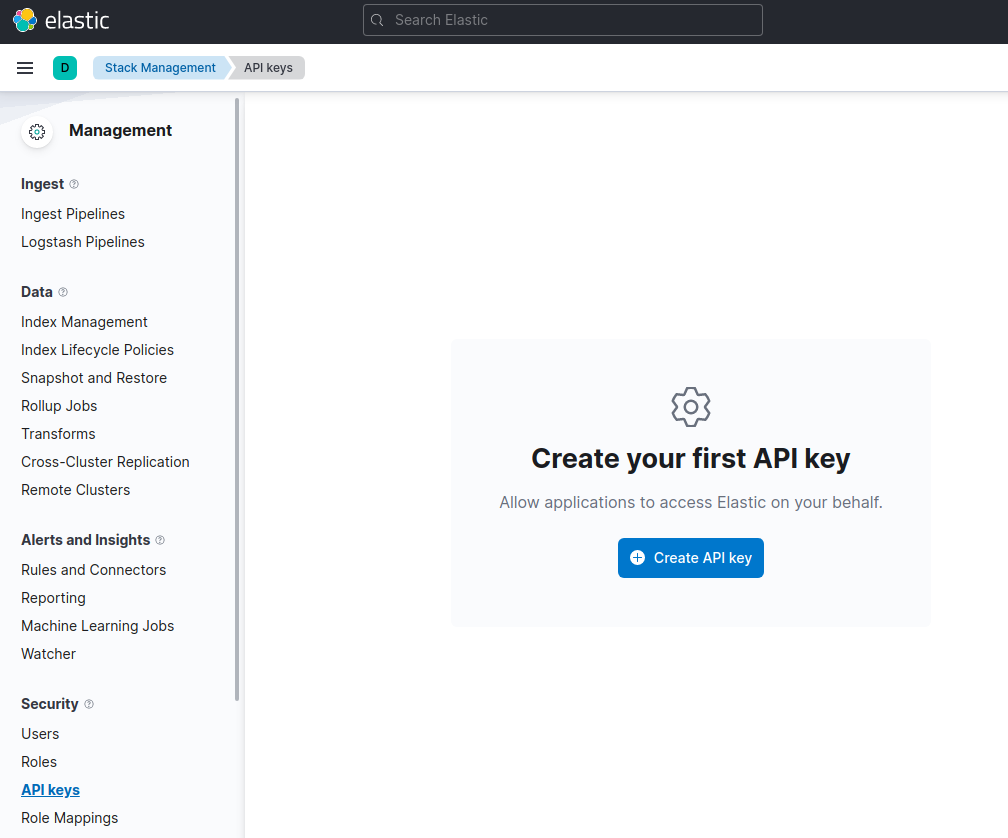
For other connection options, refer to the Connecting section.
Operations
editTime to use Elasticsearch! This section walks you through the basic, and most important, operations of Elasticsearch.
Creating an index
editThis is how you create the my_index
index:
await client.indices.create({ index: 'my_index' })
Indexing documents
editThis is a simple way of indexing a document:
await client.index({ index: 'my_index', id: 'my_document_id', document: { foo: 'foo', bar: 'bar', }, })
Getting documents
editYou can get documents by using the following code:
await client.get({ index: 'my_index', id: 'my_document_id', })
Searching documents
editThis is how you can create a single match query with the client:
await client.search({ query: { match: { foo: 'foo' } } })
Updating documents
editThis is how you can update a document, for example to add a new field:
await client.update({ index: 'my_index', id: 'my_document_id', doc: { foo: 'bar', new_field: 'new value' } })
Deleting documents
editawait client.delete({ index: 'my_index', id: 'my_document_id', })
Deleting an index
editawait client.indices.delete({ index: 'my_index' })
Further reading
edit- Use Client helpers for a more comfortable experience with the APIs.
- For an elaborate example of how to ingest data into Elastic Cloud, refer to this page.